Webhook Integration
What are Webhooks?
Webhooks are automated messages sent from apps when something happens - they’re like real-time notifications for applications. Instead of constantly checking for updates, your application receives instant notifications when events occur.
CyberBlog uses webhooks to automatically deliver newly published blog posts to your server. When a post is published, we send an HTTPS request with a JSON payload containing all the post data to your specified endpoint. This enables you to:
- Automatically publish posts to your CMS
- Sync content across multiple platforms
- Create custom workflows for content review
- Store posts in your own database
Getting Started
Create Endpoint
Set up an endpoint in your application to receive webhook requests. Your endpoint should:
- Accept POST requests
- Process JSON payloads
- Return 200 status code on success
Here’s a basic example using Next.js API routes (app/api/cyberblog/route.ts
):
import { NextResponse } from 'next/server'
export async function POST(req: Request) {
const payload = await req.json()
// Log the webhook payload
console.log('Received webhook:', payload)
// Process the webhook payload here
// ... your processing logic ...
return NextResponse.json({ received: true }, { status: 200 })
}
For security best practices and signature verification, see our Verify Webhooks guide.
Configure Webhook
- Navigate to
/dashboard/api/webhooks
- Click “Add Webhook”
- Enter your endpoint URL
- Select the website to associate with this webhook
- Save your configuration
Your endpoint URL must be publicly accessible and support HTTPS.
For testing webhooks locally during development, see our Local Testing Guide.
Test Integration
After configuring your webhook:
- A test event will be sent when you click the
Test Webhook
button in the CyberBlog webhook dashboard - Check your endpoint logs for the test payload
- Verify the signature using your signing secret
Monitor Events
Track webhook deliveries and responses in real-time:
- View delivery status
- Check payload contents
- Monitor success rates
- Retry failed deliveries
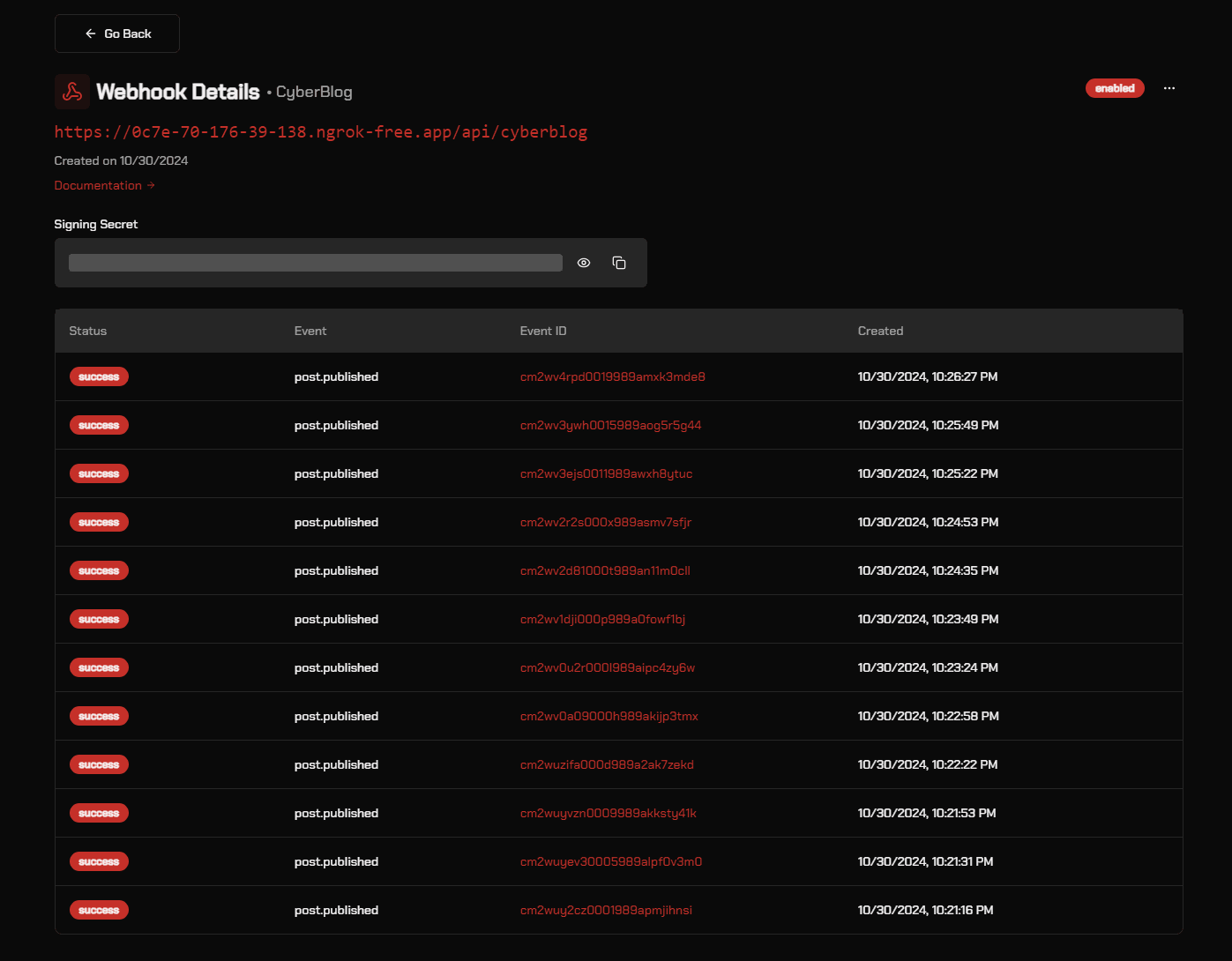
Security
All webhook requests are signed using HMAC SHA-256. Always verify the signature before processing the payload.
For implementation details, see: